Setting the Stage for Integration
Laravel, a PHP framework, excels in managing application logic and serving data, while Three.js, a JavaScript library, shines in rendering 3D content in the browser using WebGL. To illustrate their potential, we’ll create an application that dynamically generates 3D objects (a cube and a sphere) based on data imported from an Excel file.
Step 1: Preparing Laravel
Start by setting up a new Laravel project and installing the necessary packages for handling Excel files, such as Laravel Excel:composer create-project --prefer-dist laravel/laravel ThreeDLaravelVisualization composer require maatwebsite/excel
Create a model and migration for storing our Excel data:php artisan make:model DataPoint -m
In the migration file, define the structure of your data points. For simplicity, we’ll include coordinates and a type (cube or sphere):
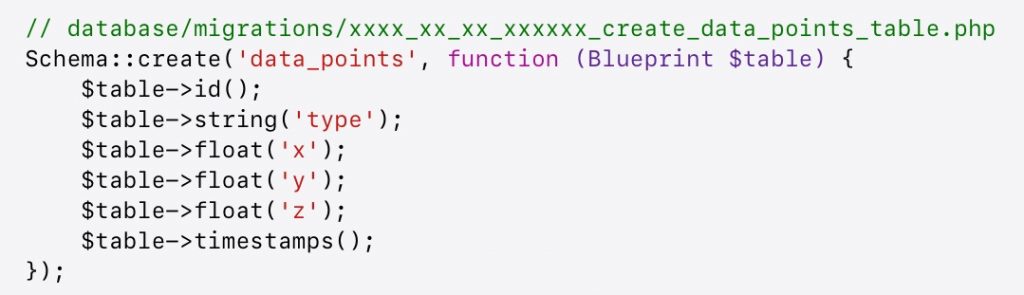
Run the migration:php artisan migrate
Step 2: Importing Data from Excel
Assuming you have an Excel file with data to import, set up Laravel Excel to read the file and populate your data_points
table. You might create an import class using Laravel Excel documentation as a guide.
Step 3: Serving Data with Laravel
Create a controller to serve your data to the front end:php artisan make:controller DataController
In DataController
, add a method to fetch data points and return them as JSON:
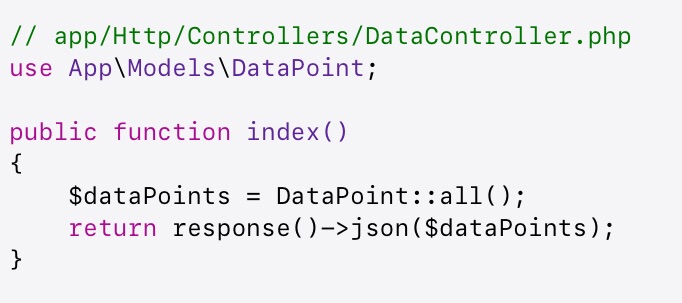
Define a route in routes/web.php
:Route::get('/data', 'DataController@index');
Crafting 3D Objects with Three.js
Now, let’s switch to the front end. In your Laravel project’s public directory, create a JavaScript file for your Three.js code, say public/js/threeApp.js
. Include this script in your main blade view:<script src="{{ asset('js/threeApp.js') }}"></script>
In threeApp.js
, initialize Three.js, set up a scene, camera, and renderer. Then, fetch your data from Laravel and create 3D objects accordingly:
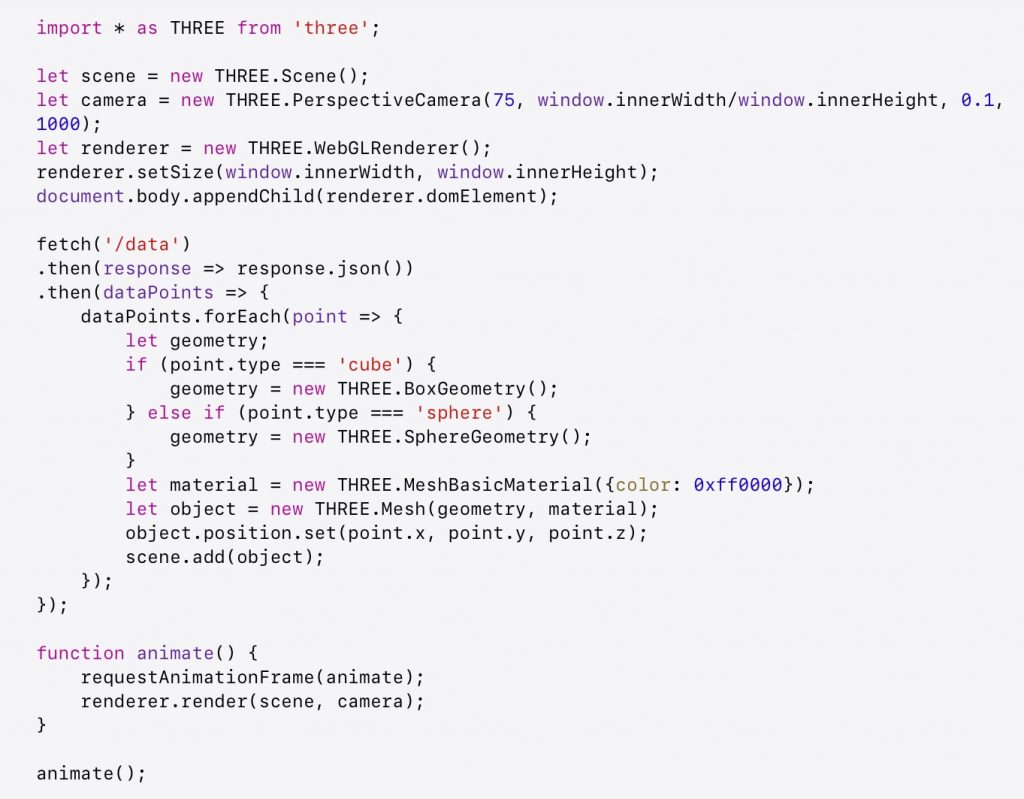
This script fetches data from Laravel, checks the type of each data point, and creates either a cube or a sphere positioned according to the data.
Conclusion
Integrating Laravel and Three.js for dynamic 3D data visualization offers a unique approach to presenting information.